Secure URL Shortener
Simple URL shortener with cybersecurity in mind.
- Next.js
- React
- TypeScript
- Safe Browsing API
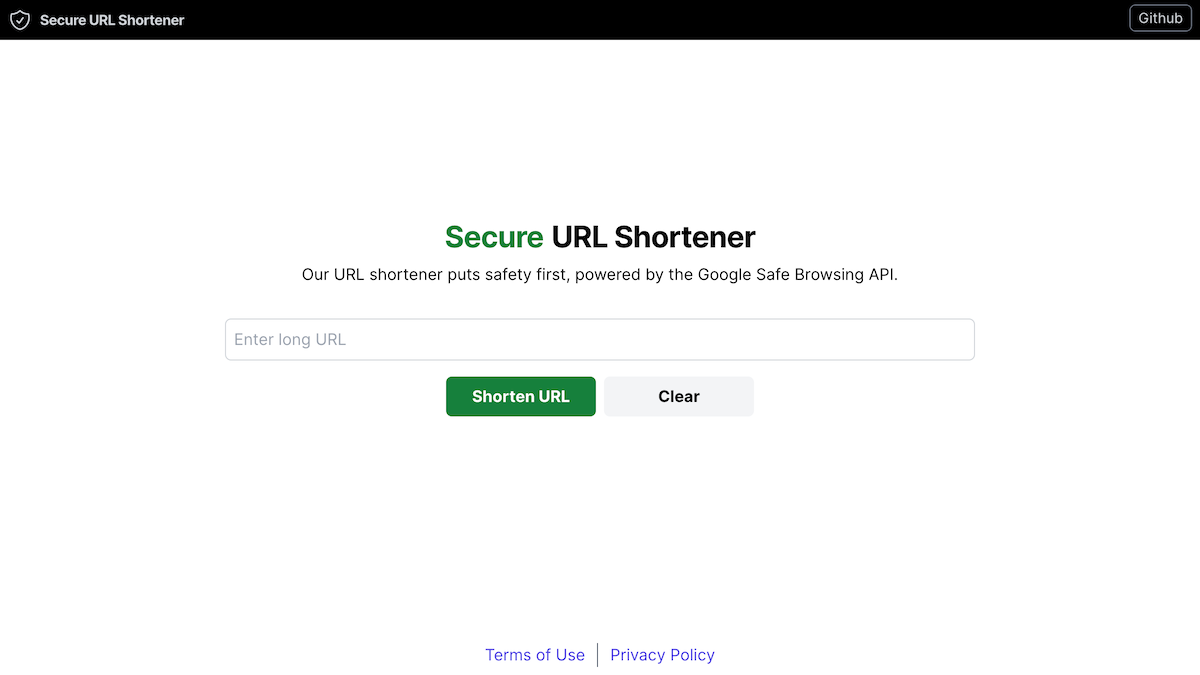
About the project
With the rise of cyber threats, ensuring the safety of URLs has become more critical than ever. That’s why I built this project in the first place: a secure URL shortener that not only generates shorter URLs but also guarantees their safety.
With our secure URL shortener, you can simply input a long URL, and our app will generate a shorter link for you to share.
How it works
Before generating the shortened URL, we check the input URL against the Safe Browsing API, ensuring that it is free from any potential threats or malicious content. This extra layer of security gives you peace of mind, knowing that the links you share are safe for both you and other people.
/**
* Function to check the given URL against the Google Safe Browsing API
* to make sure the user is not entering malicious URL.
*/
export async function checkUrlWithSafeBrowsing(url: string) {
const apiKey = process.env.SAFE_BROWSING_API_KEY;
const apiUrl = 'https://safebrowsing.googleapis.com/v4/threatMatches:find'
try {
const response: AxiosResponse<SafeBrowsingResponse> = await axios.post(apiUrl, {
client: { ... },
threatInfo: {
threatTypes: ['MALWARE', 'SOCIAL_ENGINEERING'],
threatEntryTypes: ['URL'],
threatEntries: [{ url }],
},
}, {
params: { key: apiKey },
})
const isSafe = response.data.matches && response.data.matches.length > 0
return { safe: isSafe }
} catch (e) {
return { error: `Error checking URL with Google Safe Browsing API: ${e}`
}
}
}
Once the Safe Browsing API confirmed that the URL is safe, the code will shorten the URL by simply generating a random string and assign the string to the given URL.
async function generateShortenedUrl(): Promise<string> {
const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'
const urlLength = 6
let shortened = ''
for (let i = 0; i < urlLength; i++) {
shortened += characters.charAt(Math.floor(Math.random() * characters.length))
}
// ...Check for duplication and repeat if needed...
return shortened
}
Summary
This is a simple project suitable for personal and small organizations for generating short URLs. The people who want to learn about building apps using Next.js can also use this project for learning.
You can download the source code on Github.